외로운 Nova의 작업실
안드로이드 앱 프로그래밍 - 7(사용자 이벤트 처리하기) 본문
- 키 이벤트
키 이벤트에서는 네비게이션 바의 뒤로가기 버튼과 음량버튼이 눌렸을때 함수를 호출할 수 있습니다.
onKeyDown : 키를 누른 순간의 이벤트
onKeyUp : 키를 떼는 순간의 이벤트
onKeyLongPress : 키를 오래 누르는 순간의 이벤트
package com.example.myapplication
import android.os.Bundle
import android.util.Log
import android.view.KeyEvent
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
class kakotalk : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_kakotalk)
}
override fun onKeyUp(keyCode: Int, event: KeyEvent?): Boolean {
return when (keyCode) {
KeyEvent.KEYCODE_BACK -> {
Log.d("log", "뒤로가기 버튼을 땟군요")
true
}
KeyEvent.KEYCODE_VOLUME_DOWN -> {
Log.d("log", "볼륨 다운 버튼을 땟군요")
true
}
KeyEvent.KEYCODE_VOLUME_UP -> {
Log.d("log", "볼륨 업 버튼을 땟군요")
true
}
else -> super.onKeyUp(keyCode, event)
}
}
override fun onKeyDown(keyCode: Int, event: KeyEvent?): Boolean {
return when (keyCode) {
KeyEvent.KEYCODE_BACK -> {
Toast.makeText(applicationContext, "뒤로가기 버튼을 한번더 눌르세요", Toast.LENGTH_SHORT).show()
true
}
KeyEvent.KEYCODE_VOLUME_DOWN -> {
Log.d("log", "볼륨 다운 버튼을 눌렀군요")
true
}
KeyEvent.KEYCODE_VOLUME_UP -> {
Log.d("log", "볼륨 업 버튼을 눌렀군요")
true
}
else -> super.onKeyDown(keyCode, event)
}
}
}
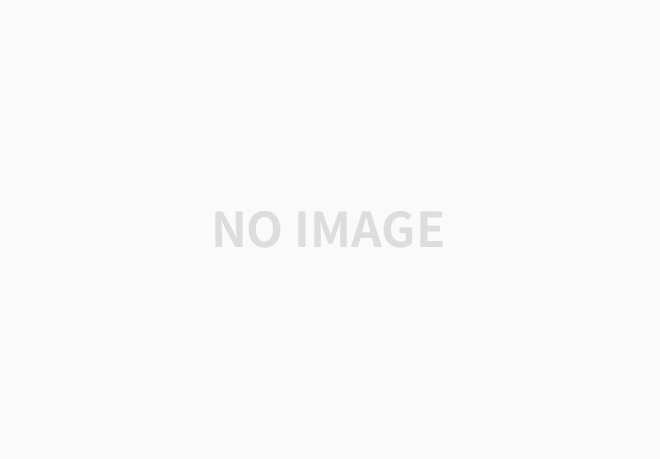
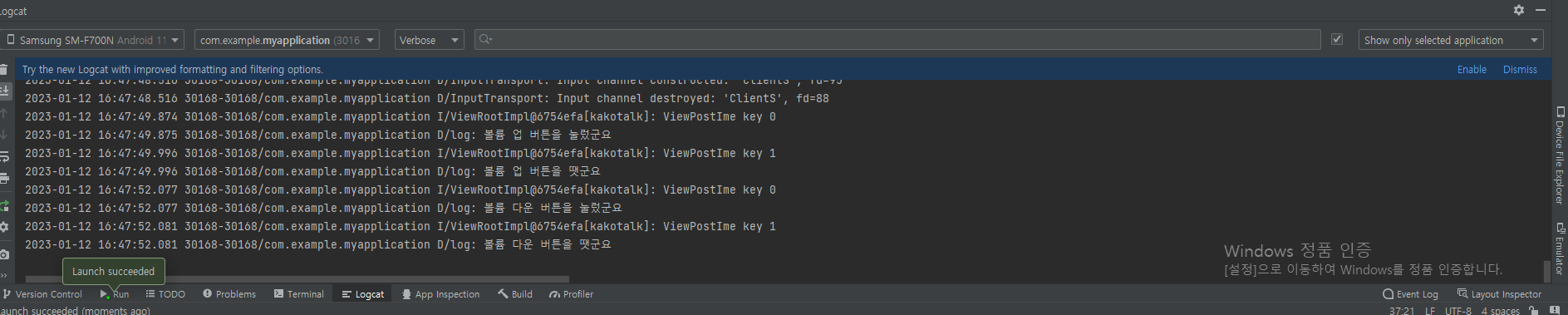
- 뷰이벤트
사용자가 하드웨어 버튼이 아닌 화면에 터치할경우 터치당한 각 뷰에서 이벤트를 별도로 제공합니다. 뷰 이벤트 처리는 이벤트 소스와 이벤트 핸들러를 리스너가 연결해줘야합니다.
- 이벤트 소스 : 이벤트가 발생한 객체
- 이벤트 핸들러 : 이벤트 발생시 실행할 로직이 구현된 객체
- 리스너 : 이벤트 소스와 이벤트 핸들러를 연결해주는 함수
<버튼 클릭 이벤트 처리>
package com.example.myapplication
import android.os.Bundle
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
import com.example.myapplication.databinding.ActivityKakotalkBinding
class kakotalk : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
val binding = ActivityKakotalkBinding.inflate(layoutInflater)
setContentView(binding.root)
binding.button1.setOnClickListener(){
Toast.makeText(applicationContext, "press button 1", Toast.LENGTH_SHORT).show()
}
binding.button2.setOnClickListener(){
Toast.makeText(applicationContext, "press button 2", Toast.LENGTH_SHORT).show()
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".kakotalk">
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:text="Button1"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:text="Button2"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
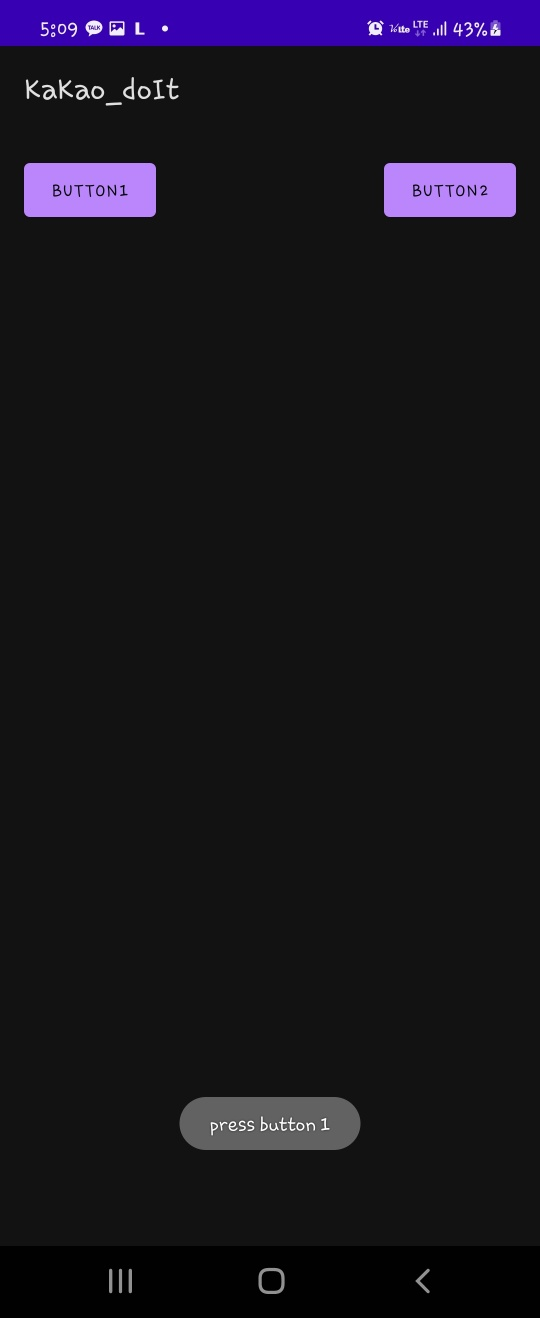
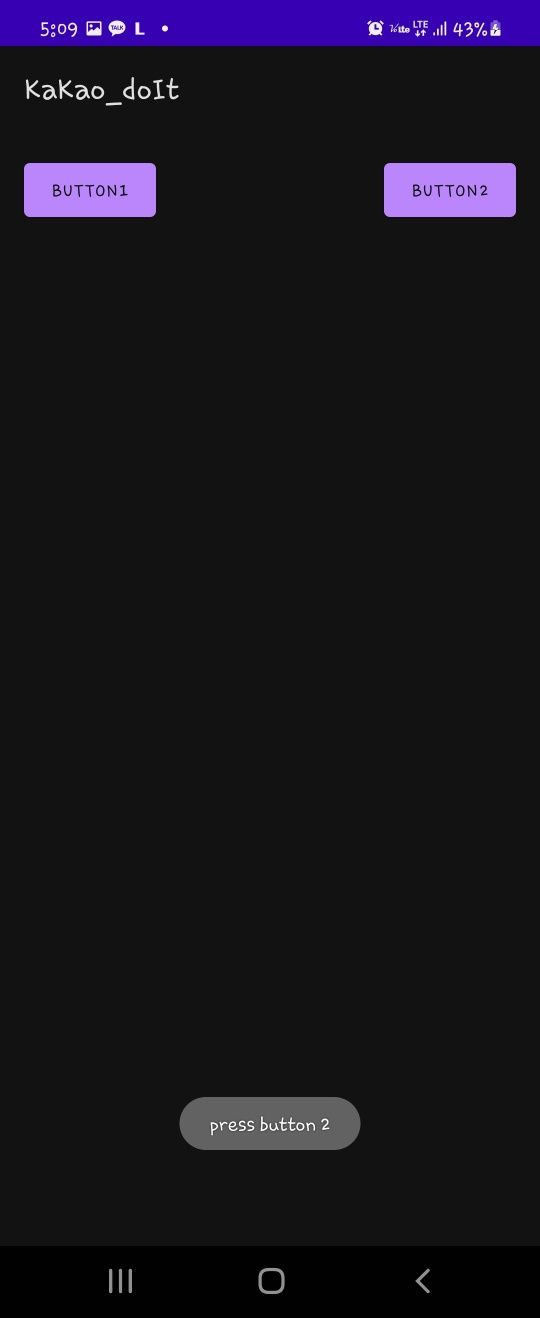
- 스톱워치 만들기
drawable>round_bottom.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle"
android:padding = "10dp">
<solid android:color="#6666FF"></solid>
<corners
android:bottomLeftRadius="30dp"
android:bottomRightRadius="30dp"
android:topLeftRadius="30dp"
android:topRightRadius="30dp"></corners>
</shape>
layout>activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<Chronometer
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/chronometer"
android:gravity="center_horizontal"
android:textSize="60dp"
android:layout_marginTop="100dp"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center_horizontal"
android:layout_alignParentBottom="true"
android:layout_marginBottom="70dp">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/startButton"
android:background="@drawable/round_botton"
android:text="start"
android:textStyle="bold"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/stopButton"
android:background="@drawable/round_botton"
android:text="stop"
android:textStyle="bold"
android:layout_marginLeft="25dp"
android:enabled="false"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/resetButton"
android:background="@drawable/round_botton"
android:text="reset"
android:textStyle="bold"
android:layout_marginLeft="25dp"
android:enabled="false"/>
</LinearLayout>
</RelativeLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.kt
.
package com.example.stopwatch
import android.os.Bundle
import android.os.SystemClock
import android.view.KeyEvent
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
import com.example.stopwatch.databinding.ActivityMainBinding
class MainActivity : AppCompatActivity() {
var pauseTime = 0L
var initime = 0L
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
val binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
binding.startButton.setOnClickListener(){
binding.chronometer.base = SystemClock.elapsedRealtime() + pauseTime
binding.chronometer.start()
binding.startButton.isEnabled = false
binding.stopButton.isEnabled = true
binding.resetButton.isEnabled = true
}
binding.stopButton.setOnClickListener(){
pauseTime = binding.chronometer.base - SystemClock.elapsedRealtime()
binding.chronometer.stop()
binding.startButton.isEnabled = true
binding.stopButton.isEnabled = false
binding.resetButton.isEnabled = true
}
binding.resetButton.setOnClickListener() {
pauseTime = 0L
binding.chronometer.stop()
binding.chronometer.base = SystemClock.elapsedRealtime()
binding.startButton.isEnabled = true
binding.stopButton.isEnabled = false
binding.resetButton.isEnabled = false
}
}
override fun onKeyDown(keyCode: Int, event: KeyEvent?): Boolean {
return when (keyCode) {
KeyEvent.KEYCODE_BACK -> {
if(System.currentTimeMillis() - initime > 3000){
Toast.makeText(applicationContext,"한번더 뒤로가기 버튼을 눌러주세요", Toast.LENGTH_SHORT)
initime = System.currentTimeMillis()
return true
}
else return true
}
else -> super.onKeyDown(keyCode, event)
}
}
}
실행화면

'Programming > Kotlin - Android' 카테고리의 다른 글
안드로이드 앱 프로그래밍 - 9(퍼미션 설정하기) (0) | 2023.01.16 |
---|---|
안드로이드 앱 프로그래밍 - 8(리소스의 종류와 특징) (0) | 2023.01.13 |
안드로이드 앱 프로그래밍 - 6(뷰를 배치하는 레이아웃) (0) | 2023.01.11 |
안드로이드 앱 프로그래밍 - 5(뷰를 이용한 화면 구성) (0) | 2023.01.09 |
안드로이드 앱 프로그래밍 - 4(코틀린의 유용한 기법) (0) | 2023.01.09 |